Wednesday, 6 November 2024
Send OTP Using C# MVC5
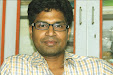
Monday, 21 August 2023
CaptchaMvc Mvc5 | C# ASP.Net
CaptchaMvc Mvc5 Using C# ASP.Net Visual Studio 2019
CAPTCHA stands for "Completely Automated Public Turing test to tell Computers and Humans Apart". It is a test used to distinguish between humans and computers. CAPTCHAs are often used to prevent automated spam and abuse.
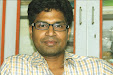
Friday, 10 March 2023
CCAvenue Integration with C# ASP.NET MVC
CCAvenue integration with complete step-by-step code for C# ASP.NET MVC Pattern.
Process Login
- Signup with CCavenue and get the CcAvenueMerchantId,CcAvenueWorkingKey, and CcAvenueAccessCode. To get this code please refer to the ccavenue documentation or use youtube because this information is available easily. So I am going to leave this to make this post smaller.
- Download the Integration kit named ASP.Net_Kit_Version_2.0.
- Drop an email to service@ccavenue.com with your website URL because CCAvenue entertains only register URLs sent by users by mail.
- CCavenue provides a form with mandatory and optional parameter then encrypt user input and send it to the server.
Step 1
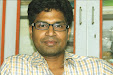
Thursday, 15 December 2022
FormsAuthentication C# ASP.NET MVC
FormsAuthentication
FormsAuthentication class from System.Web.Security is used to authenticate a user whether login or not. FormsAuthentication class has many functions to check the user login and it's very easy to implement. Follow the code given below
Views
<h2>Login</h2>
<form method="post">
<input type="text" name="UserName" placeholder="User Name" required /><br /><br />
<input type="password" name="Password" placeholder="Password" required /><br /><br />
<input type="submit" value="Login" />
@if
(Request.QueryString["msg"] != null)
{
<br /><br />
<span style="color:red">@Request.QueryString["msg"]</span>
}
</form>
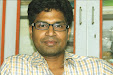
Thursday, 10 November 2022
Make PDF from hyperlink | ASP.NET C#
This post will help you to create a PDF file from a URL in ASP.Net C#. Follow the steps given below. We will save a PDF file on the server then after viewing the PDF file, the file will be deleted.
Step 1 :
Download Select.HtmlToPdf.NetCore package from NuGet.
Goto Tools Menu>Nuget Package Manager > Manage Nuget Package for Solution
Step 2 :
Step 3
function createPDF(pageurl)
{
$.ajax({
url: '/Common/htmltopdf/',
async: true,
dataType: "json",
type: "GET",
contentType: 'application/json; charset=utf-8',
data: { url: pageurl},
success: function (data) {
DelPDF('/upload/print/' + $.trim(invid) + '.pdf');
window.location.href = '/upload/print/' + $.trim(invid) + '.pdf';
}
});
}
function DelPDF(path)
{
$.ajax({
url: '/Common/DeleteCommonFile?path=' + path,
type: "POST",
contentType: false, // Not to
set any content header
processData: false, // Not to process data
success: function (result) {
//Update Extension
if (result.sMessage == "1")
{
}
else
alert(result.sMessage);
},
error: function (abc) {
alert(abc.statusText);
}
});
}
Step 4
Controller C# code
public JsonResult htmltopdf(string url,string inv)
{
// instantiate
a html to pdf converter object
HtmlToPdf converter = new HtmlToPdf();
// create a new
pdf document converting an url
PdfDocument doc =
converter.ConvertUrl(url);
// save pdf
document
doc.Save(Server.MapPath("~/upload/print/" + inv + ".pdf"));
// close pdf
document
doc.Close();
return Json(new { sMessage = "11"}, JsonRequestBehavior.AllowGet);
}
[HttpPost]
public JsonResult DeleteCommonFile(string path)
{
try
{
string filename = Server.MapPath(path);
if (System.IO.File.Exists(filename))
{
System.IO.File.Delete(filename);
}
return Json(new {
sMessage = "1",
JsonRequestBehavior.AllowGet });
}
catch (Exception ex)
{
return Json(new {
sMessage = ex.Message, JsonRequestBehavior.AllowGet });
}
}
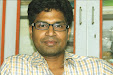
Thursday, 13 January 2022
Multiple file upload by form post ASP.NET C# MVC
Multiple file upload by form post ASP.NET C# MVC
This post will let you upload multiple image files through a single form post using the C# ASP.Net MVC platform.
Key Point
- Upload multiple image files with a new name
- Save a associate record and file name will be newly created record identity field value
- No need to save the image name in the database because the file name will start with a record ID and end with a text.
- Best for putting student, employee documents without any hassle.
HTML Code :
<form method="post" enctype="multipart/form-data">
@Html.AntiForgeryToken()
<p class="m-0">
Photo
<input type="file" name="Photo" id="Photo" accept="image/x-png,image/jpeg" />
<br />
Adhaar
<input type="file" name="Adhaar" id="Adhaar" accept="image/x-png,image/jpeg" />
<br />
Pan
<input type="file" name="Pan" id="Pan" accept="image/x-png,image/jpeg" />
<br />
<input type="checkbox" id="chkconfirm" /> I
provide my consent. I will follow the Tangentweb term condition and policy.
<br /> <br />
<input type="submit" value="Save" class="button
button-primary button-wide-mobile" onclick="return filecheck()" />
</p>
</form>
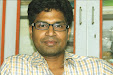
Thursday, 4 November 2021
Fill Menu items in partial view from database without using java script in C# ASP.NET MVC
Fill Menu items in partial view from the database without using javascript in C# ASP.NET MVC.
When there is a need to load menu items from the database, then you may have many options like load it from javascript. But the best load time is when you use Razor collaborate with C#. This post will help you to do the same. Follow the step-by-step instructions. I Guarantee that response time is the lowest than any other approach.
Model
public class MenuModel
{
public string ID { get; set; }
public string MenuName { get; set; }
}
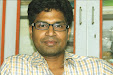
Friday, 22 October 2021
Read INI file ASP.Net MVC C#
Install ini reference
> nuget install ini-parseror
PM> Install-Package ini-parser
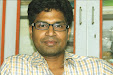
Friday, 15 October 2021
Save file to server without java script C# ASP.Net
In this post, you will learn, How to upload an image file to a server without using javascript or jquery. The file will be upload using C#. Unique File name is generated with datetime string , you implement your logic for the file name. Given code is applicable in both C# MVC and ASP.Net both.
Name Space
using System.IO;
HTML
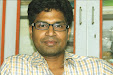
Saturday, 12 June 2021
Stripe Integration with C# ASP .NET MVC Visual Studio 2013
Stripe payment gateway integration in ASP.NET C# MVC using Visual Studio 2013
Stripe payment gateway is simple but documentation for integration is very complicated. Current integration documentation is written for .Net core and if your project is made with visual studio 2013, then it would be very complicated to integrate because no documentation is available for the version of VS 2013. So I have made this post which will help you to strip integration with ASP.Net MVC using C#.
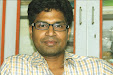
Monday, 7 June 2021
Install NuGet Package in Visual Studio 2013 | Microsoft ASP .NET
Install NuGet Package in Microsoft Visual Studio 2013 ASP.NET
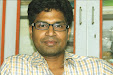
Monday, 17 May 2021
Solved : Application of .NET Technology January 2020
Solved : Application of .NET Technology January 2020
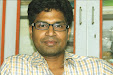
Tuesday, 20 April 2021
Custom Error message | Hide Yellow Screen Of Death (YSOD) page in ASP .Net
Yellow Screen Of Death (YSOD)
Setting in Webconfg file
<system.web>
<compilation debug="true" targetFramework="4.7.2" />
<httpRuntime targetFramework="4.7.2" />
<trust level="Full" />
<customErrors mode="RemoteOnly"
defaultRedirect="~/Shared/Error.cshtml" />
</system.web>
Show error in custom error file
@model System.Web.Mvc.HandleErrorInfo
<div class="container">
<h1>Error.</h1>
<h2>An
error occurred while processing your request.</h2>
<h3 style="color:red"><p style="color:red"> @Model.Exception.Message</p></h3>
<br /><br />
</div>
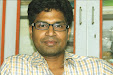